The Anqu Method Pattern
The anqu method pattern for Java suggests to have a central method to access a @NamedQuery or @NamedNativeQuery. In short the pattern proposes the following construction for a given query: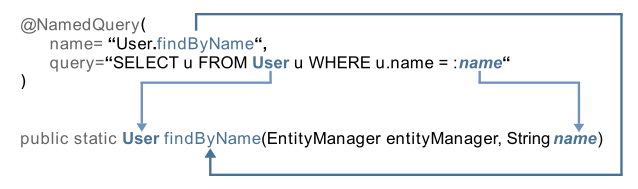
You may already be using this pattern in some variation - you could call it common sense. What is not shown is the method body containig the glue code to execute the query. It is cumbersome to implement and the API to use is one of the most general APIs you can think of. Nearly every parameter is either just Object or at best String. Writing that code is error-prone.
Building the bridge between the textual nature of the @NamedQuery statement and the modeled Java world early reduces risks and enables you to use compiler based tooling to increase coding comfort.
Driven by this the anqu method pattern consists of three principles:
If you use Eclipse as IDE, the anqu method plug-in can generate the Java method in a few mouse clicks. You get a specialized, typed, already implemented, and unit-tested API for every query. The plug-in helps to implement all steps mentioned above and has addtional features to increase productivity.
Please note that in the example above the EntityManager is provided as a parameter of a static method. Though this is optional/configurable, this variation is considered to be the earliest transition you can come up with.